React 18 Design Patterns And Best Practices Carlos Santana Roldaacuten Pdf Free Download
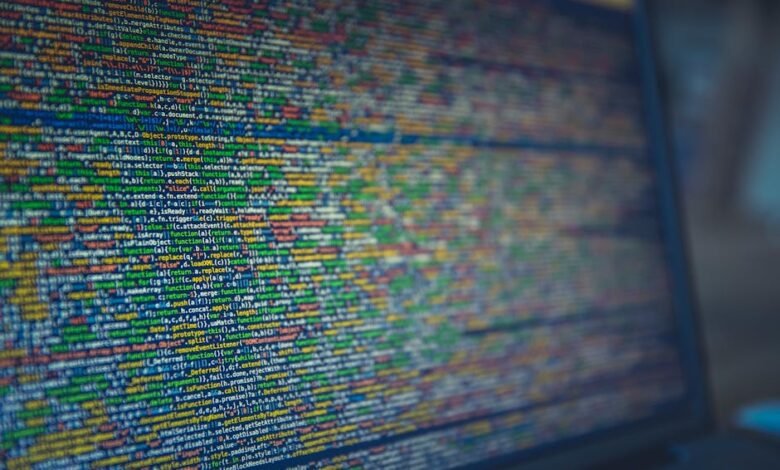
Hey there, fellow developers! Are you diving into the awesome world of React 18? It’s packed with cool new features that can make your apps faster and more efficient. If you’re searching for a free PDF download of “React 18 Design Patterns and Best Practices” by Carlos Santana Roldán, you’re probably looking for some guidance on how to use React 18 the right way. While I can’t provide a direct link to a free PDF of that specific resource (copyright laws, you know!), I can definitely help you understand the key design patterns and best practices in React 18. Think of me as your friendly guide to navigating this exciting new version!
Let’s be honest, learning a new framework or library can be overwhelming. There’s so much to take in! That’s where design patterns come in. They’re like tried-and-true recipes for solving common problems in your code. And best practices? They’re the tips and tricks that experienced developers use to write clean, maintainable, and performant code. Together, they make you a more effective and efficient developer. So, let’s get started!
Key Design Patterns in React 18
React 18 introduces some exciting changes, and understanding how to leverage design patterns is crucial for building scalable and maintainable applications. Here are a few essential ones:
The Provider Pattern (Context API)
The Provider pattern, often implemented using React’s Context API, is all about making data available to multiple components without having to pass it down through props at every level. Imagine you have a theme (light or dark) that needs to be accessible to every component in your application. Instead of passing the theme down through each component individually, you can use the Context API. The Provider component wraps your app, and any component within that wrapper can access the theme data using the `useContext` hook. This simplifies your code and makes it much easier to manage global state.
The Compound Component Pattern
The Compound Component pattern is fantastic for creating reusable UI components that work together seamlessly. Think of a set of tabs, where each tab is a separate component, but they all need to coordinate with each other to show the correct content. Instead of exposing a complex API for each individual tab, you create a parent component that manages the state and behavior of all the tabs. The individual tab components then implicitly share this state, making the whole system easier to use and more predictable.
The Render Props Pattern
The Render Props pattern is a technique for sharing code between React components using a prop whose value is a function. This function returns a React element. Essentially, the component with the render prop delegates the rendering logic to the function passed in as a prop. This is particularly useful when you need to share behavior or data between components without tightly coupling them. It offers great flexibility because the parent component controls what is rendered.
The Higher-Order Component (HOC) Pattern
Higher-Order Components (HOCs) are functions that take a component as an argument and return a new, enhanced component. This pattern is commonly used to add extra functionality to existing components, such as authentication, logging, or data fetching. While HOCs are still useful, React hooks often provide a more elegant and composable solution for many of the same use cases.
React 18 Best Practices
Now that we’ve covered some key design patterns, let’s move on to some best practices for writing efficient and maintainable React 18 code:
Embrace Concurrent Rendering
React 18 introduces concurrent rendering, which allows React to work on multiple tasks at the same time. This can significantly improve the performance of your application, especially when dealing with large and complex components. One of the key features of concurrent rendering is interruptible rendering. This means that React can pause, resume, or even abandon a rendering task if a more important task comes along. This helps prevent the UI from becoming unresponsive, even during heavy computations.
Use Transitions for UI Updates
Transitions are a new feature in React 18 that allow you to mark certain state updates as non-urgent. This tells React to prioritize more important updates, such as user input, over these less critical updates. This can lead to a smoother and more responsive user experience. For example, you might use a transition for updating a search results list, allowing React to prioritize typing in the search bar over rendering the new results.
Optimize Component Rendering with `useMemo` and `useCallback`
React components re-render whenever their props or state change. However, sometimes a component might re-render even when its props and state haven’t actually changed. This can lead to unnecessary performance overhead. The `useMemo` and `useCallback` hooks can help you optimize component rendering by memoizing values and functions, respectively. `useMemo` allows you to cache the result of a computation, so it’s only recalculated when its dependencies change. `useCallback` allows you to create memoized versions of functions, so they only change when their dependencies change. This prevents unnecessary re-renders of child components that depend on these functions.
Keep Components Small and Focused
Just like with any programming language, it’s important to keep your React components small and focused. Each component should have a single responsibility, making it easier to understand, test, and maintain. If a component starts to become too large or complex, consider breaking it down into smaller, more manageable sub-components.
Write Comprehensive Tests
Testing is an essential part of any software development project, and React is no exception. Write unit tests for your components to ensure they behave as expected. Use integration tests to verify that different parts of your application work together correctly. Testing helps catch bugs early, reduces the risk of regressions, and makes it easier to refactor your code with confidence.
Leverage Code Splitting
Code splitting is a technique for breaking your application into smaller chunks that can be loaded on demand. This can significantly improve the initial load time of your application, especially for large and complex projects. React provides built-in support for code splitting using the `React.lazy` function and the `Suspense` component. By splitting your code into smaller chunks, you can reduce the amount of JavaScript that needs to be downloaded and parsed initially, leading to a faster and more responsive user experience.
Frequently Asked Questions
What are the biggest advantages of using React 18?
React 18 brings improved performance through concurrent rendering, better user experience with transitions, and new hooks for more efficient state management. It makes building responsive and complex UIs much smoother.
How does concurrent rendering in React 18 work?
Concurrent rendering allows React to work on multiple UI updates at the same time, prioritizing important tasks like user input. This makes your app feel faster and more responsive because React can pause and resume rendering tasks as needed.
When should I use transitions in React 18?
Use transitions for non-urgent UI updates, like filtering a list or updating a chart. This tells React to prioritize other tasks, like responding to user clicks or typing, resulting in a smoother user experience.
So, while I couldn’t provide that specific PDF, I hope this overview of React 18 design patterns and best practices has been helpful. Remember, the best way to learn is by doing! Experiment with these patterns and practices in your own projects, and don’t be afraid to explore the official React documentation for more in-depth information. Happy coding!